VoiceBase’s Developer API offers application companies an open platform for scalable & secure voice analytics.
Custom Analytics
Get the results you want for the solutions you need, using our robust analytics toolkit.
Flexible Integrations
Build integrations to wherever your data is and wherever you want your results to end up.
PCI Certified Platform
Process your voice data through VoiceBase’s PCI Level 1 DSS Certified platform.
The Developer API enables SaaS applications to extract voice analytics across any audio, video, or text content. Our platform was built with flexibility in mind. Get the results you want, with full access to all your data. Process massive volumes and never worry about downtime or system breaches.Â
How it works
The VoiceBase API is easy to use. Extract your data from anywhere and get a simple JSON output for your analytic results.
?php
$bearertoken = ‘your-token’;
$configuration = ‘your-json-configuration’;
$metadata = ‘your-json-metadata’;
$mediaUrl = ‘your-media-url’;
$url = “https://apis.voicebase.com/v3/media”;
$headers = array(
   ‘Authorization: Bearer ‘ . $bearertoken,
‘Content-Type: multipart/form-data’
);
set_time_limit(0);
$curl = curl_init();
$params = array(
“configuration” => $configuration,
“metadata” => $metadata,
“mediaUrl” => $mediaUrl
);
curl_setopt($curl, CURLOPT_POST, 1);
curl_setopt($curl, CURLOPT_HTTPHEADER, $headers);
curl_setopt($curl, CURLOPT_URL, $url);
curl_setopt($curl, CURLOPT_POSTFIELDS, $params);
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
 echo “cURL Error #:” . $err;
} else {
 echo $response;
}​
?>
curl --location --request POST
'https://apis.voicebase.com/v3/media' \
--header 'Content-Type: multipart/form-data' \
--header 'Authorization: Bearer $TOKEN' \
--form 'mediaUrl=https://www.example.com/sample.wav' \
--form 'configuration={
  "speechModel": { "language": "en-US" }}'
#python v3-postman-python-post-mediaUrl.py
import requests
url = “https://apis.voicebase.com/v3/media/”
media_file = ‘https://www.example.com/sample.wav’
payload= {‘mediaUrl’: media_file}
payload[‘configuration’] = (‘configuration.json’, ‘{“speechModel” : {
“language” : “en-US”} }’,’application/json’)
headers = {
    ‘Authorization’: “Bearer $TOKEN”
    }
response = requests.post(url, files=payload, headers=headers)
print(response.text)
<?php
$bearertoken = 'your-token';
$configuration = 'your-configuration';
$metadata = 'your-json-metadata';
$mediapath = '@sample.wav';
$url = "https://apis.voicebase.com/v3/media";
$headers = array(
  'Authorization: Bearer ' . $bearertoken,
  'Content-Type: multipart/form-data'
);
set_time_limit(0);
$curl = curl_init();
$params = array(
"configuration" => $configuration,
"metadata" => $metadata,
"media" => $mediapath
);
curl_setopt($curl, CURLOPT_POST, 1);
curl_setopt($curl, CURLOPT_HTTPHEADER, $headers);
curl_setopt($curl, CURLOPT_URL, $url);
curl_setopt($curl, CURLOPT_POSTFIELDS, $params);
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
 echo "cURL Error #:" . $err;
} else {
 echo $response;
}
?>
curl --location --request POST 'https://apis.voicebase.com/v3/media/' \
--header 'Authorization: Bearer $TOKEN' \
--form 'configuration={
   "speechModel":{
      "language":"en-US"
   }
}' \
--form 'media=@/Media/sample.wav'
#python v3-postman-python-post-multipart-file.py import requests url = "https://apis.voicebase.com/v3/media" media_file = open('sample.wav','rb') payload = {'media': ('sample.wav', media_file, 'audio/mpeg')} payload['configuration'] = ('configuration.json', '{"speechModel" : { "language" : "en-US"} }','application/json') headers = { Â Â Â Â 'Authorization': "Bearer $TOKEN" Â Â Â Â } response = requests.post(url, files=payload, headers=headers) print(response.text)
Extract Voice Data From Anywhere
VoiceBase can ingest your audio, video, or text content from anywhere. Whether that be from your IVR system, social media messages, help platforms, or more.
Use the Language You Know
Use the language you already know and don’t worry about anything but uploading your files to get results.
Upload Using a Mediaurl or the File
Upload your voice data via mediaUrl or the actual voice file with a simple request across the most common coding languages.
Get JSON Results Fast
Your analytics will come out as a simple JSON format result. This open format allows for an easy understanding of your voice data for rapid insights. Get one response when your data has been uploaded, and another with the final transcripts.
Simple yet Powerful Transcripts
Get your transcript results with the accompanying metadata. This example shows a stereo speaker example of a transcript. When each speaker’s turn begins and ends is included, as well as who was speaking.Â
{
  "_links": {
    "self": {
      "href": "/v3/media/1ef668ce-0feb-484e-95f1-797e446ca889"
    },
    "progress": {
      "href": "/v3/media/1ef668ce-0feb-484e-95f1-797e446ca889/progress"
    },
    "metadata": {
      "href": "/v3/media/1ef668ce-0feb-484e-95f1-797e446ca889/metadata"
    }
  },
  "formatVersion": "3.0.1",
  "mediaId": "1ef668ce-0feb-484e-95f1-797e446ca889",
  "status": "accepted",
  "dateCreated": "2017-10-13T20:48:04.494Z",
  "metadata": {},
  "mediaContentType": "audio/x-aiff",
  "length": 14965
}
{
  "mediaId": "bc14632d-e81b-4673-992d-5c5fb6573fb8",
  "status": "finished",
  "dateCreated": "2017-06-22T19:18:49Z",
  "dateFinished": "2017-06-22T19:19:27Z",
  "mediaContentType": "audio/x-wav",
  "length": 10031,
  "transcript": {
     "words":[
        {
           "p":1,
           "s":2200,
           "c":1.0,
           "e":2350,
           "w":"agent",
           "m":"turn"
        },
        {
           "p":2,
           "s":2200,
           "c":0.537,
           "e":2300,
           "w":"Hello"
        },
        {
          "p":3,
          "s":2300,
          "c":1.0,
          "e":2300,
          "w":".",
          "m":"punc"
        },
        {
           "p":4,
           "s":2400,
           "c":0.1,
           "e":2550,
           "w":"caller",
           "m":"turn"
        },
        {
           "p":5,
           "s":2400,
           "c":0.975,
           "e":2500,
           "w":"Hi"
        }
     ]
  }
}
Custom Voice Analytics
Full access to the power behind VoiceBase’s full stack of analytic features. Customize speech-to-text transcription, redact sensitive info, and categorize your calls in an optimal way for your business.
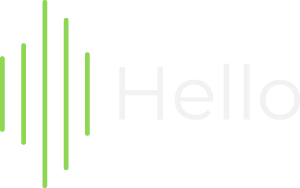
Speech-To-text
Get custom text transcripts that include stereo speaker ID, custom vocab, word-by-word timestamps, and PCI Redaction.Â
PCI Redaction
Automatically detect and redact PCI/PII information from your voice data using VoiceBase’s PCI DSS Level 1 Certified platform.
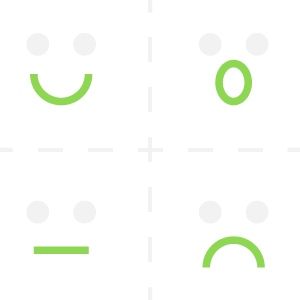
Metrics
Dive into the metrics from your conversations such as overtalk, silence, and sentiment for a deep understanding of what is being said.
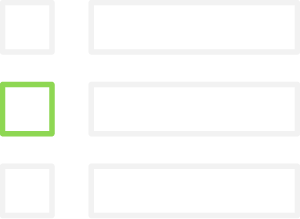
Categorization
Take advantage of dozens of categories from pre-built libraries or build custom categories that make sense for your business needs.
Predictions
Detect & predict complex behavior, like customer churn, across all of your voice data with high accuracy using machine learning.
Text Analytics
Analyze conversations chat, email, CRM, and support text data for a complete view of customer interactions.
Our Platform
Built with flexibility in mind.
Scalable
VoiceBase’s API was built on a hybrid public/private cloud architecture. Whether your application requires a thousand minutes/month of processing or a hundred million minutes, VoiceBase has you covered.
Secure
Enterprise-grade security has been a key feature of VoiceBase’s design from the very beginning. Every aspect of both the system and its hosting environment was designed to isolate and protect customer data.
Reliable
Our system is highly distributed, fully redundant, and self-healing. We deliver consistent uptime, with no downtime windows for maintenance or system upgrades. Rely on us to get the results you want with no issues.
Core Technology
The technology powering VoiceBase’s award-winning analytics
Speech Engine
The VoiceBase speech engine is based on a large vocabulary speech recognition (LVSR) platform that utilizes deep learning neural networks to best determine what was said. VoiceBase has developed features such as custom vocabulary, parallel processing, and number formatting in order to provide industry-leading accuracy and analytical results.
Analytics
In addition to the transcript, VoiceBase automatically surfaces keywords, key phrases, and topics from recordings using proprietary automatic speech recognition (ASR) algorithms and customer input. This enables businesses to identify trends, perform competitive analysis and better track each customer’s journey. VoiceBase also enables complex queries with a SQL-like Query language built for voice analytics.
Machine Learning
The API platform uses machine learning to perform predictive analysis of spoken information to automatically classify complex and subjective events. Businesses can utilize Predictive Insights to improve sale conversion rates, detect pre-churn, increase efficiency, and automate processes such as quality compliance measures.
Certifications
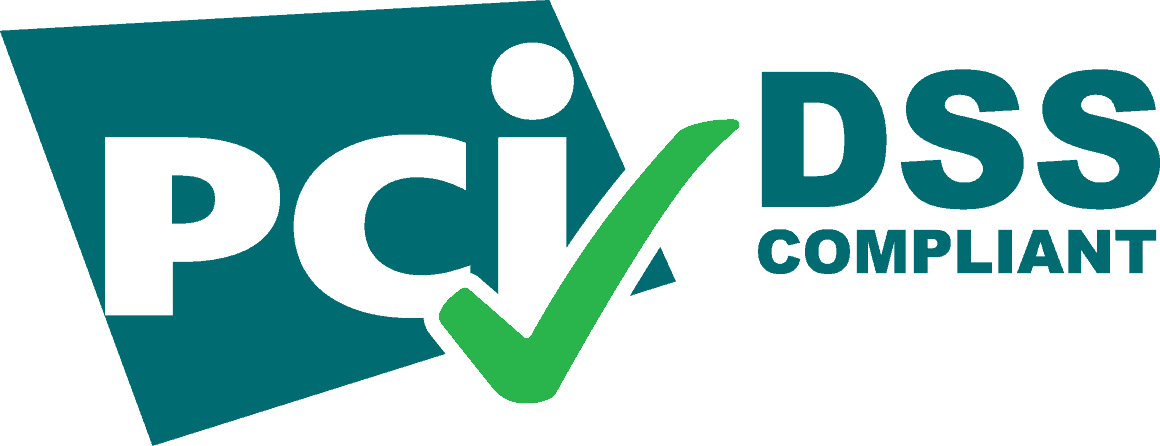
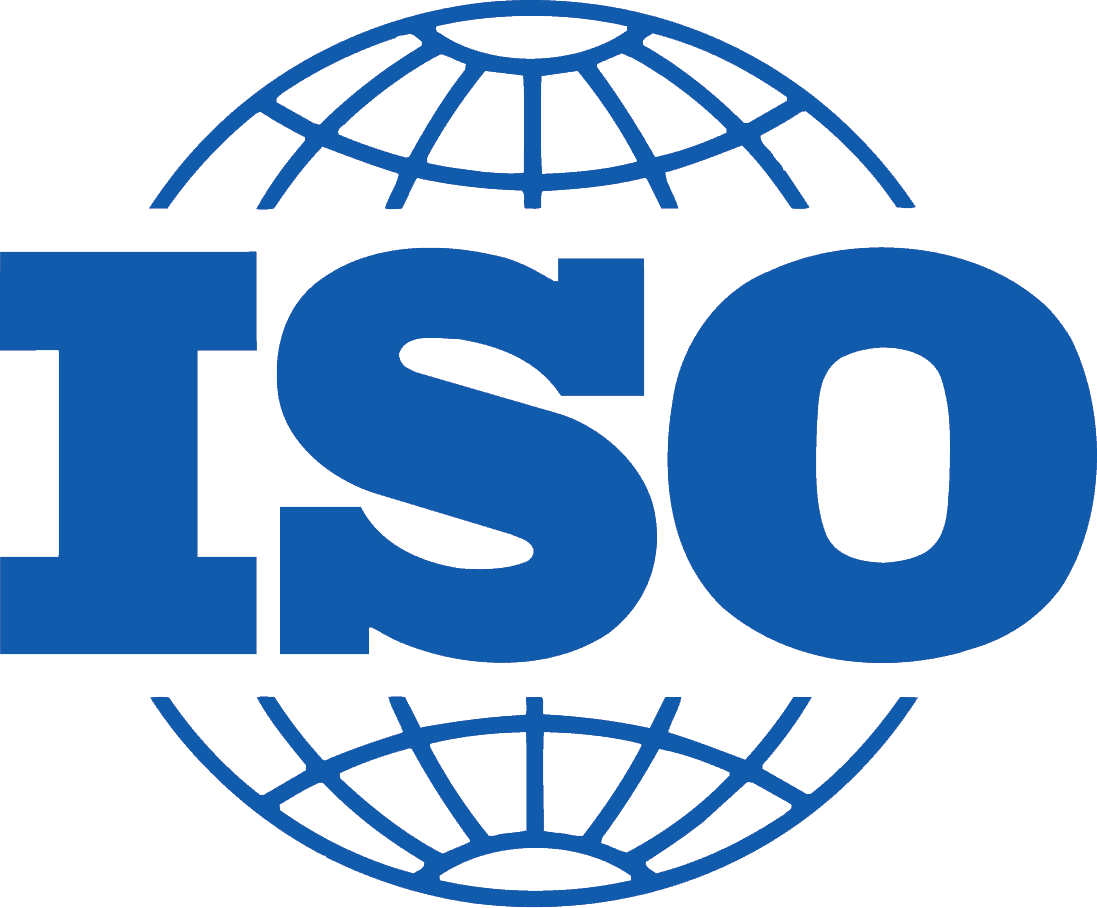
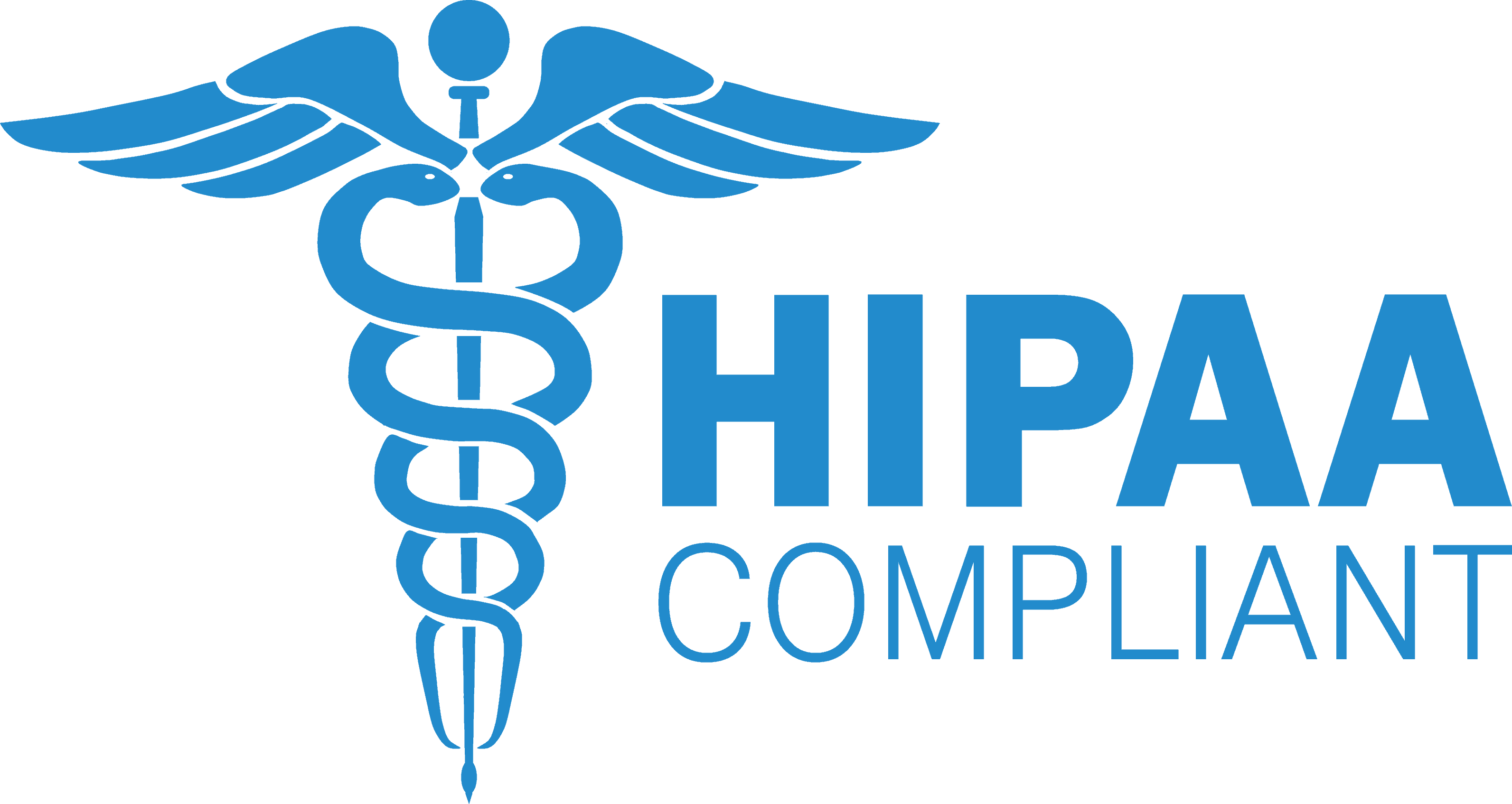
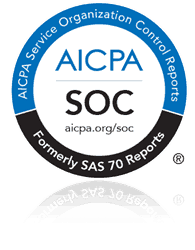
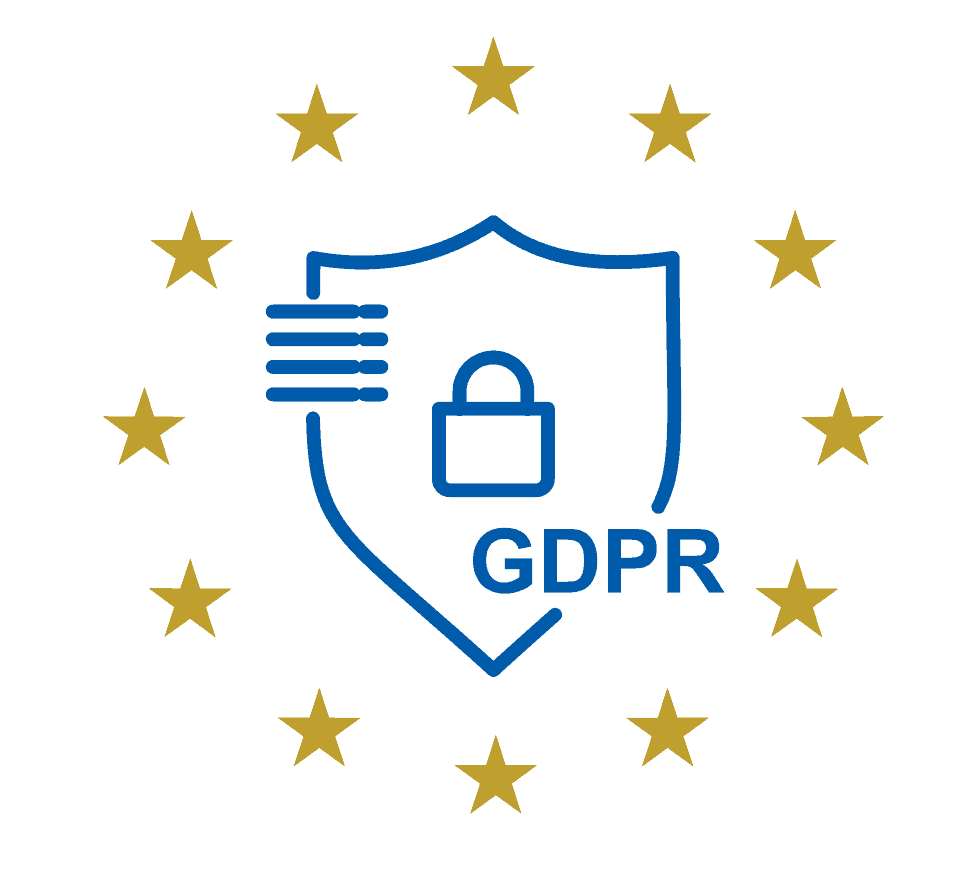
VoiceBase Developer API
The powerful API enabling companies to perform flexible and reliable analysis across large volumes of voice data.
Already Have Access to the API?
Access all of your analytics in one place with VoiceBase’s dev portal.